选择框 - checkbox
# 勾选框控件: checkbox
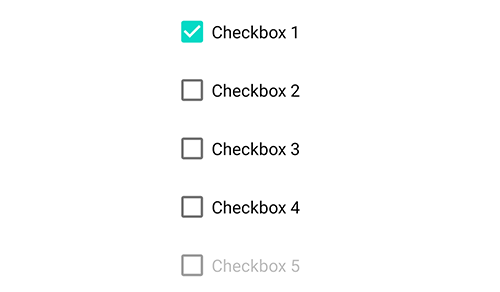
## text
checkbox控件的文本,显示在勾选框后面。实际上checkbox控件继承于text和button控件,它们的属性也可以用在checkbox上,比如textSize, textStyle。
## checked
设置checkbox的选中状态,当checked="true"为选中选项框,否则为未选中选项框。可以通过attr方法动态设置勾选框的状态。比如:$ui.checkbox1.attr("checked", "true")。
## enabled
设置复选框是否是启用的,若enabled="false"则为禁用状态,显示未灰色,无法交互。
## isChecked()
返回控件是否选中。
返回值为值为true或false
```js
log(ui.objId.isChecked())
```
## setChecked(value)
设置控件选中。
value的值为true或false
```js
ui.objId.setChecked(true)
```
## 自定义控件-模块-配置勾选框(APP自带例子)
```js
//这个自定义控件是一个勾选框checkbox,能够保存自己的勾选状态,在脚本重新启动时能恢复状态
var PrefCheckBox = (function() {
//继承至ui.Widget
util.extend(PrefCheckBox, ui.Widget);
function PrefCheckBox() {
//调用父类构造函数
ui.Widget.call(this);
//自定义属性key,定义在配置中保存时的key
this.defineAttr("key");
}
PrefCheckBox.prototype.render = function() {
return (
<checkbox />
);
}
PrefCheckBox.prototype.onFinishInflation = function(view) {
view.setChecked(PrefCheckBox.getPref().get(this.getKey(), false));
view.on("check", (checked) => {
PrefCheckBox.getPref().put(this.getKey(), checked);
});
}
PrefCheckBox.prototype.getKey = function() {
if(this.key){
return this.key;
}
let id = this.view.attr("id");
if(!id){
throw new Error("should set a id or key to the checkbox");
}
return id.replace("@+id/", "");
}
PrefCheckBox.setPref = function(pref) {
PrefCheckBox._pref = pref;
}
PrefCheckBox.getPref = function(){
if(!PrefCheckBox._pref){
PrefCheckBox._pref = storages.create("pref");
}
return PrefCheckBox._pref;
}
ui.registerWidget("pref-checkbox", PrefCheckBox);
return PrefCheckBox;
})();
module.exports = PrefCheckBox;
```
## 自定义控件-使用配置勾选框(APP自带例子)
```js
"ui";
var PrefCheckBox = require('./自定义控件-模块-配置勾选框.js');
ui.layout(
<vertical>
<pref-checkbox id="perf1" text="配置1"/>
<pref-checkbox id="perf2" text="配置2"/>
<button id="btn" text="获取配置"/>
</vertical>
);
ui.btn.on("click", function(){
toast("配置1为" + PrefCheckBox.getPref().get("perf1"));
toast("配置2为" + PrefCheckBox.getPref().get("perf2"));
});
```
### 保存与读取选择框状态的例子
```js
"ui";
ui.layout(
<vertical >
<Switch id="is1"/>
</vertical>
)
ui.is1.on("click", function() {
if (ui.is1.isChecked()) {
log(1)
files.write("///sdcard/i.dat", "true")
} else {
files.write("///sdcard/i.dat", "false")
}
})
if (files.read("///sdcard/i.dat") == "true") {
ui.is1.setChecked(true)
} else {
ui.is1.setChecked(false)
}
```
### 修改checkbox大小
```js
'ui';
// 大柒
ui.layout(
<vertical>
<checkbox id='cb' text='改变checkbox大小' />
</vertical>
);
let view = ui.cb;
//获取CheckBox默认样式
let drawable = view.getButtonDrawable();
//隐藏其默认样式
view.setButtonDrawable(new android.graphics.drawable.ColorDrawable(colors.TRANSPARENT));
//移动组件并调整其大小。前两个参数指定左上角的新位置,后两个参数指定新的大小。必须设置图片大小,否则不显示
drawable.setBounds(0, 0, 100, 100);
//设置drawable显示在checkbox左侧
view.setCompoundDrawables(drawable, null, null, null);
```